Create Default Profile Image Dynamically from First and Last Name in PHP
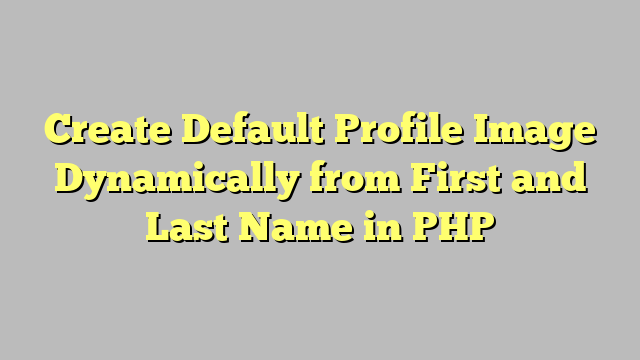
Table of Content
Default image is used as the profile image when a user registers on a website. This default image is displayed as the profile image until the user changes their profile image. Generally, the same image is used as the default profile image for all users. If you want to separate default images for each user, they need to be changed dynamically.
In this code snippet, we will show how to dynamically create a default profile picture from first and last name in PHP. Since the image uses the first letter of the first and last name, the profile image will be different for each user and relevant to their name.
Get first letter of first and last name using PHP
First, we need to split the full name into first name and last name and generate short characters from the username.
- Take the first letter of the first and last name.
- Combine two letters to create a short character full name.
<?php // Full name of the user $user_full_name = 'Demo User'; $full_name_arr = explode(" ", $user_full_name); $full_name_arr_end = end($full_name_arr); $firstWord = !empty($full_name_arr[0])?$full_name_arr[0]:''; $lastWord = !empty($full_name_arr_end[0])?$full_name_arr_end[0]:''; $charF = !empty(mb_substr($firstWord, 0, 1))?mb_substr($firstWord, 0, 1):''; $charL = !empty(mb_substr($lastWord, 0, 1))?mb_substr($lastWord, 0, 1):''; $shortChar = $charF.$charL; ?>
Create an HTML element with the first letters of the first and last name:
Print short characters from the default image in HTML.
<div class="profile-image"> <?php echo trim($shortChar); ?> </div>
Define CSS to display the default profile image:
Apply CSS to the HTML element selector (profile image) to make it look like an image.
<style> .profile-image { width: 120px; height: 120px; border-radius: 50%; background: #512DA8; font-size: 35px; color: #fff; text-align: center; line-height: 120px; margin: 20px 0; } </style>
Thanks for codexworld.com
Comments